Upgrade & Secure Your Future with DevOps, SRE, DevSecOps, MLOps!
We spend hours scrolling social media and waste money on things we forget, but wonโt spend 30 minutes a day earning certifications that can change our lives.
Master in DevOps, SRE, DevSecOps & MLOps by DevOps School!
Learn from Guru Rajesh Kumar and double your salary in just one year.
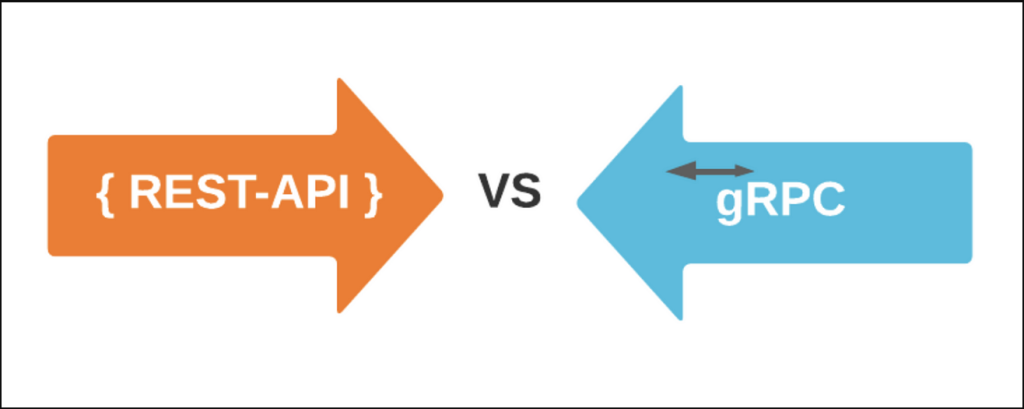
Hereโs a comprehensive post titled “Microservices Communication: REST vs. gRPC vs. Event-Driven Architecture” with expanded content for each section:
Understanding Microservices Communication
In a microservices architecture, different services need to communicate with each other to share data, trigger actions, and coordinate workflows. Effective communication between microservices is crucial to ensure the reliability, performance, and scalability of the entire system. There are various ways to enable this communication, each with its own strengths and trade-offs. Three common approaches are REST, gRPC, and Event-Driven Architecture.
In this post, weโll compare these three communication styles, exploring their major features, benefits, and best-use cases. By the end of this guide, youโll be able to choose the right communication method for your microservices architecture based on your project requirements.
Key Features of Microservices Communication Approaches
- REST: A stateless, resource-based communication style using HTTP and widely used in web applications.
- gRPC: A high-performance, HTTP/2-based protocol for service-to-service communication, using Protobuf for serialization.
- Event-Driven: A communication style where services communicate asynchronously via events, typically through message brokers.
1. REST: The Traditional Choice for Microservices Communication
REST (Representational State Transfer) has been the go-to approach for building web APIs for many years, and it continues to be widely used for communication between microservices. RESTful APIs rely on HTTP methods (GET, POST, PUT, DELETE) to interact with resources over the web.
Key Features of RESTful Communication
- Human-Readable: REST uses JSON or XML for message format, which is easy to read, debug, and work with for developers.
- Stateless: Every request in REST is independent, meaning no session state is stored on the server. This simplifies server-side architecture but requires clients to manage any necessary state.
- Platform-Independent: REST works over standard HTTP, which is supported by virtually all programming languages and platforms, making it a highly interoperable choice for microservices.
- Easy to Integrate: REST APIs are simple to integrate with other systems or external services, as they follow widely accepted standards.
- Caching Support: HTTP’s built-in support for caching can help improve the performance of REST APIs, reducing the load on backend services.
When to Use REST for Microservices Communication
- Legacy Systems: If you’re working with legacy systems or third-party services that already expose RESTful APIs, itโs easier to stick with REST.
- Simplicity: When simplicity and ease of integration are top priorities, REST is a good choice due to its widespread use and straightforward design.
- Interoperability: If you need to support a variety of clients, from mobile apps to web browsers, RESTโs HTTP-based architecture makes it suitable for handling diverse client types.
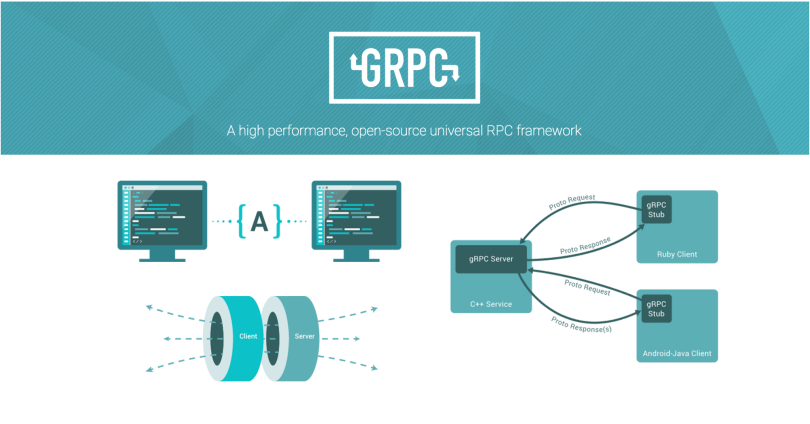
2. gRPC: High-Performance Communication for Microservices
gRPC (gRPC Remote Procedure Call) is a high-performance, language-agnostic communication protocol developed by Google. gRPC uses Protocol Buffers (Protobuf) for serialization and HTTP/2 for transport, making it faster and more efficient than traditional REST.
Key Features of gRPC
- HTTP/2-Based: gRPC leverages HTTP/2, which supports multiplexing, persistent connections, and efficient data transfer, improving performance compared to RESTโs HTTP/1.1.
- Protobuf Serialization: gRPC uses Protocol Buffers (Protobuf) for message serialization, which is faster and more compact than JSON or XML used by REST.
- Bidirectional Streaming: gRPC supports bidirectional streaming, allowing both the client and the server to send messages independently and continuously, making it ideal for real-time applications.
- Code Generation: gRPC provides code generation tools, enabling developers to automatically generate client and server code from service definitions, which speeds up development.
- Strongly Typed: With Protobuf, the service definitions are strongly typed, reducing errors and providing clearer APIs.
When to Use gRPC for Microservices Communication
- High-Performance Systems: For systems where performance and low-latency communication are crucial, such as real-time applications, gRPC is a superior choice.
- Inter-Service Communication: When microservices need to communicate internally within the same organization, gRPC offers faster communication with lower overhead than REST.
- Streaming and Real-Time Data: gRPCโs support for bidirectional streaming makes it ideal for applications like video streaming, chat applications, or real-time data pipelines.
- Strong API Contracts: If you need strongly typed interfaces, gRPCโs use of Protobuf offers a better structure and reduces issues related to schema management.
3. Event-Driven Architecture: Asynchronous Communication for Scalability
Event-Driven Architecture (EDA) involves communication where services produce and consume events asynchronously. In this model, services communicate by publishing events to a message broker (e.g., Kafka, RabbitMQ), which then routes those events to other services interested in the events.
Key Features of Event-Driven Architecture
- Asynchronous Communication: In EDA, services donโt wait for a direct response; instead, they react to events. This decouples services and increases system resilience.
- Loose Coupling: Services in an event-driven system are loosely coupled, as they donโt need to know about each otherโs implementation or status. They only need to understand the events they are producing or consuming.
- Scalability: Since services are loosely coupled and communicate via events, itโs easy to scale them independently based on demand. This makes EDA highly suitable for large-scale systems.
- Event Sourcing: Events can be used as the source of truth, meaning that they store the state transitions of the system, enabling easier rebuilding of the system state or auditing.
- Real-Time Processing: Event-driven architectures are ideal for systems that require real-time processing of incoming data, such as IoT or logging systems.
When to Use Event-Driven Architecture
- Decoupled Microservices: If you need to decouple services and allow them to operate independently, event-driven architecture provides a highly flexible solution.
- Asynchronous Workflows: If your system needs to handle asynchronous workflows, such as processing background tasks or integrating with external systems, event-driven communication is ideal.
- Scalable Systems: For systems that require high scalability, particularly when you expect bursts of traffic or need to scale components independently, EDA provides the flexibility to handle those demands efficiently.
- Real-Time Data Processing: If you are dealing with real-time events, like user activities or IoT sensor data, EDA enables you to process data as it arrives without blocking or waiting for responses.
4. Comparing REST, gRPC, and Event-Driven Architecture
Now that weโve explored the features of REST, gRPC, and Event-Driven Architecture, let’s compare them directly to understand which one is best suited for different use cases.
Comparison of Communication Styles
Feature | REST | gRPC | Event-Driven Architecture |
---|---|---|---|
Protocol | HTTP/1.1, JSON/XML | HTTP/2, Protobuf | Message Brokers (e.g., Kafka) |
Communication Style | Synchronous | Synchronous / Asynchronous | Asynchronous |
Latency | Higher due to HTTP/1.1 overhead | Lower due to HTTP/2 and Protobuf | Depends on message broker latency |
Scalability | Moderate, harder to scale | High, scales well for internal communication | Excellent for highly scalable systems |
Use Cases | Web APIs, public services | Real-time applications, internal communication | Decoupled systems, real-time data processing |
Complexity | Simple to implement, easy to integrate | Complex to implement and maintain | Can be complex to manage events and consistency |
Performance | Lower performance for large payloads | High performance and low overhead | Scalable but requires overhead for event processing |
5. Choosing the Right Communication Method for Your Microservices
The choice between REST, gRPC, and Event-Driven Architecture depends largely on your projectโs specific requirements, such as scalability, performance, and the type of services being built.
When to Choose REST
- Public APIs: REST is ideal for exposing public APIs to external clients or third-party systems. Its simplicity, widespread support, and human-readable format make it a great choice.
- Interoperability: When working with a variety of clients (web, mobile, third-party systems), RESTโs reliance on HTTP ensures easy communication across different platforms.
When to Choose gRPC
- High-Performance Internal Communication: For systems where microservices communicate internally, especially in real-time or low-latency scenarios, gRPC is ideal.
- Strongly Typed APIs: If you require strongly typed interfaces and automatic code generation, gRPC with Protobuf is a great fit.
When to Choose Event-Driven Architecture
- Decoupled Systems: For highly decoupled systems where microservices need to operate independently and asynchronously, event-driven architecture excels.
- Scalable, Real-Time Applications: Event-driven systems work well for real-time data processing, event-sourcing, and applications that require high scalability.
Selecting the Right Communication Style
Choosing the right communication method for your microservices is essential for ensuring that your system meets its performance, scalability, and reliability requirements. REST is perfect for simpler, stateless APIs, gRPC is ideal for high-performance, internal communication, and Event-Driven Architecture excels in scalable, decoupled, and real-time systems.
By carefully evaluating your needsโsuch as latency, message size, communication patterns, and system complexityโyou can select the communication method that aligns best with your projectโs goals and maximize the benefits of a microservices architecture.
Leave a Reply