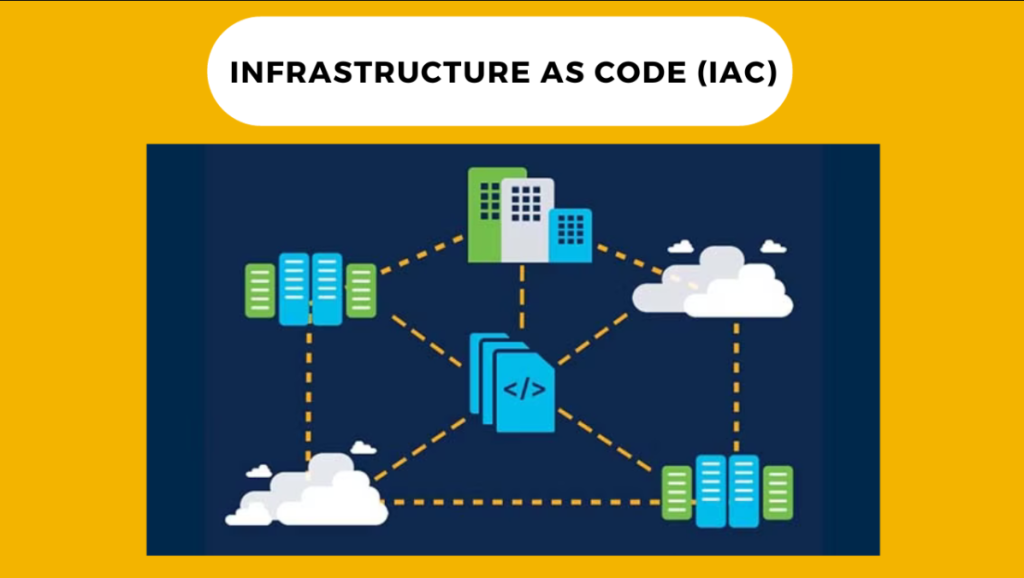
Here’s a comprehensive post titled “Getting Started with Infrastructure as Code: A Beginner’s Guide” with expanded content for each section:
What is Infrastructure as Code (IaC)?
Infrastructure as Code (IaC) is a modern practice that allows developers and system administrators to define and manage infrastructure through code, rather than manual processes. This practice enables the automation of provisioning, configuring, and managing cloud environments. IaC provides consistency, repeatability, and transparency by treating infrastructure as software. By using code to define infrastructure, teams can automate deployment, configuration, and scaling of infrastructure resources, significantly improving efficiency and reducing the risk of human error.
In this beginner’s guide, we’ll explore what IaC is, its key benefits, and how to get started with this essential DevOps practice. We’ll also dive into the core concepts, tools, and technologies you’ll need to begin working with IaC and how it integrates into modern cloud environments.
Key Features of IaC
- Automation: IaC automates the creation, configuration, and management of infrastructure, reducing the need for manual intervention.
- Version Control: By defining infrastructure in code, IaC configurations can be stored in version control systems, enabling rollback, auditability, and tracking of changes.
- Consistency: IaC ensures that infrastructure environments are provisioned identically across multiple environments (e.g., development, staging, production).
- Scalability: IaC enables scalable infrastructure management, allowing you to easily adjust your resources as your needs grow or shrink.
- Improved Collaboration: By treating infrastructure as code, development and operations teams can collaborate more effectively using the same tools and processes.
1. Key Concepts in Infrastructure as Code
Before diving into practical steps, it’s important to understand the core concepts of IaC. These principles guide how infrastructure is defined, provisioned, and managed through code. Understanding these concepts will provide a solid foundation for your IaC journey.
Core Concepts in IaC
- Declarative vs. Imperative Approaches:
- Declarative (Desired State Configuration): You describe the desired end state of your infrastructure (e.g., “I want 3 web servers”), and the IaC tool ensures that the infrastructure matches this state.
- Imperative (Action-Based Configuration): You define the specific steps to reach the desired infrastructure (e.g., “Install web server, configure port 80”).
- Idempotence: IaC should be idempotent, meaning that running the same configuration multiple times should result in the same infrastructure state without causing unintended side effects.
- Provisioning and Configuration Management: IaC tools automate the provisioning (creating resources like servers, networks, etc.) and configuration (setting up services, software, and settings) of cloud resources.
- State Management: IaC tools often maintain the state of the infrastructure, allowing them to track changes and ensure that infrastructure is provisioned according to the desired specifications.
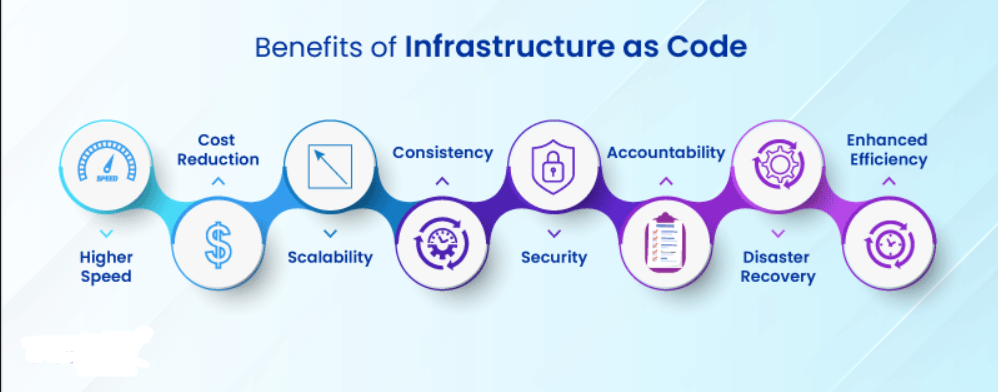
2. Benefits of Using Infrastructure as Code
IaC provides several advantages, particularly in managing cloud environments. Understanding these benefits is key to appreciating why IaC is becoming an essential practice for DevOps teams.
Benefits of IaC
- Speed and Efficiency: IaC automates infrastructure deployment, reducing manual work and speeding up the process of provisioning and configuring infrastructure.
- Consistency Across Environments: By using the same IaC scripts across all environments, you ensure that your staging, testing, and production environments are identical, which helps avoid configuration drift and bugs that arise from environment inconsistencies.
- Cost Savings: Automation leads to more efficient resource allocation and management, which can reduce operational costs by optimizing the usage of infrastructure.
- Versioning and Rollback: IaC allows infrastructure to be versioned, providing the ability to track changes and easily roll back to previous versions if something goes wrong.
- Improved Collaboration: Developers, system administrators, and operations teams can work together more effectively by managing infrastructure through code stored in version control systems like Git.
- Scalability and Flexibility: IaC tools enable you to scale infrastructure easily, add resources dynamically, or modify configurations based on workload requirements.
3. Tools and Technologies for Getting Started with IaC
Several tools and platforms are available to help you get started with IaC. These tools simplify infrastructure provisioning, management, and scaling by automating key processes and integrating seamlessly with cloud providers.
Popular IaC Tools
- Terraform: An open-source IaC tool that allows you to define infrastructure in code using a high-level configuration language. Terraform is widely used due to its support for multiple cloud providers (e.g., AWS, Google Cloud, Azure) and its declarative approach to infrastructure management.
- AWS CloudFormation: An IaC service provided by AWS for defining and provisioning AWS resources. CloudFormation templates are written in JSON or YAML and allow you to define the entire infrastructure in a single file.
- Ansible: A powerful automation tool that can be used for configuration management, application deployment, and infrastructure provisioning. Ansible uses YAML files to define infrastructure and is known for its simplicity and agentless architecture.
- Azure Resource Manager (ARM) Templates: A Microsoft Azure-native IaC service that allows you to define and manage resources on Azure using JSON-based templates.
- Pulumi: A newer IaC tool that allows you to define infrastructure using general-purpose programming languages like JavaScript, TypeScript, Python, and Go, offering a more flexible and developer-friendly approach compared to traditional declarative tools.
4. Writing and Running Your First IaC Script
Getting started with IaC involves writing simple configuration files that describe the infrastructure you need. These files can then be executed by IaC tools to provision the resources automatically.
Writing and Running a Basic IaC Script
- Step 1: Choose an IaC Tool: Start by selecting an IaC tool. If you’re working with AWS, AWS CloudFormation or Terraform might be a good choice. For multi-cloud environments, Terraform is widely recommended.
- Step 2: Define Infrastructure in Code: Write the configuration for your infrastructure using the tool’s language. For example, in Terraform, you might define an EC2 instance with a configuration file that looks like this:
resource "aws_instance" "example" { ami = "ami-0c55b159cbfafe1f0" instance_type = "t2.micro" }
- Step 3: Initialize the IaC Tool: Use the tool’s initialization command to download necessary plugins and set up your environment. For Terraform, use
terraform init
. - Step 4: Apply the Configuration: Run the command to apply the configuration and provision the resources. For Terraform, this would be
terraform apply
. - Step 5: Review and Manage Infrastructure: After provisioning, you can use the IaC tool to modify, scale, or destroy the infrastructure, keeping everything in sync with your code.
5. Managing IaC at Scale
As your infrastructure grows, managing multiple configurations and resources across different environments becomes a challenge. Fortunately, IaC tools offer several features to manage large-scale infrastructure with ease.
Scaling with IaC
- Modularization: Break your IaC configurations into smaller, reusable modules to manage large infrastructures effectively. For example, with Terraform, you can define reusable modules for common resources like VPCs, subnets, and security groups.
- Environment-Specific Configurations: Use variables, workspaces, or different configuration files for different environments (e.g., development, staging, production). This ensures that you can manage distinct settings for each environment while maintaining a single source of truth.
- State Management: Use remote state backends to manage IaC state files, allowing teams to collaborate on the same infrastructure and preventing conflicts. For Terraform, this could mean using Terraform Cloud or AWS S3 as a backend to store state.
- Version Control: Store your IaC scripts in Git repositories to track changes, collaborate with team members, and ensure that all configurations are versioned and auditable.
6. Best Practices for IaC Security
Security is a crucial aspect of any infrastructure, and IaC is no different. Misconfigurations in IaC can lead to security vulnerabilities, so it’s essential to incorporate security practices throughout your IaC workflow.
Best Security Practices for IaC
- Use Secrets Management: Never hardcode sensitive information such as API keys, credentials, or tokens directly in IaC scripts. Use secrets management tools like AWS Secrets Manager, HashiCorp Vault, or Azure Key Vault to securely store and manage secrets.
- Apply the Principle of Least Privilege: Define the minimum permissions required for each resource. Avoid broad IAM roles or excessive permissions in your IaC configurations.
- Review and Enforce Security Policies: Integrate security scanning tools into your CI/CD pipeline to detect misconfigurations and security vulnerabilities in IaC files before deployment. Tools like Checkov, Snyk, and TFLint can scan IaC scripts for common security issues.
- Automate Compliance Checks: Ensure that your IaC configurations comply with industry standards and regulations by implementing policy-as-code frameworks like Terraform Sentinel or Open Policy Agent (OPA).
- Continuous Monitoring and Auditing: Use cloud-native tools (e.g., AWS Config, Azure Security Center) to continuously monitor and audit the compliance of your deployed infrastructure, ensuring it remains secure and aligned with policies.
Embracing IaC for Modern Infrastructure Management
Infrastructure as Code (IaC) has revolutionized the way we manage cloud infrastructure. By enabling automation, consistency, and scalability, IaC provides a robust foundation for modern DevOps practices and continuous deployment. Getting started with IaC might seem daunting at first, but with the right tools, best practices, and a solid understanding of the concepts, you can quickly begin provisioning and managing your cloud resources through code.
Whether you’re automating a small web application or scaling an enterprise-level infrastructure, IaC empowers teams to build, manage, and maintain environments more efficiently and securely. By following best practices and adopting IaC tools, you can ensure that your infrastructure is always aligned with your desired configuration, compliant with security policies, and adaptable to future changes.